100. Same Tree
Same Tree
Given the roots of two binary trees p
and q
, write a function to check if they are the same or not.
Two binary trees are considered the same if they are structurally identical, and the nodes have the same value.
Example 1:
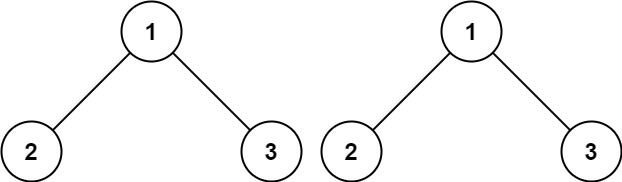
Input: p = [1,2,3], q = [1,2,3] Output: true
Example 2:
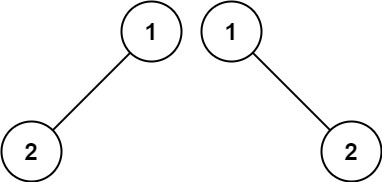
Input: p = [1,2], q = [1,null,2] Output: false
Example 3:
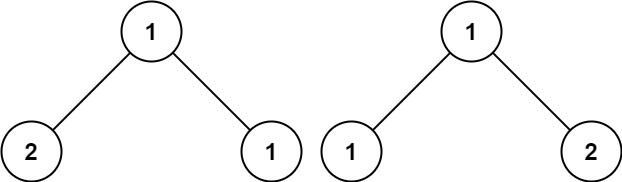
Input: p = [1,2,1], q = [1,1,2] Output: false
Constraints:
- The number of nodes in both trees is in the range
[0, 100]
. -104 <= Node.val <= 104
/** * Definition for a binary tree node. * function TreeNode(val, left, right) { * this.val = (val===undefined ? 0 : val) * this.left = (left===undefined ? null : left) * this.right = (right===undefined ? null : right) * } */ /** * @param {TreeNode} p * @param {TreeNode} q * @return {boolean} */ var isSameTree = function(p, q) { if (!p && !q) return true if (!p || !q || p.val !== q.val) return false return (isSameTree(p.left, q.left) && isSameTree(p.right, q.right)) };
That’s all folks! In this post, we solved LeetCode problem 100. Same Tree
I hope you have enjoyed this post. Feel free to share your thoughts on this.
You can find the complete source code on my GitHub repository. If you like what you learn. feel free to fork 🔪 and star ⭐ it.
In this blog, I have tried to collect & present the most important points to consider when improving Data structure and logic, feel free to add, edit, comment, or ask. For more information please reach me here
Happy coding!
Comments
Post a Comment