124. Binary Tree Maximum Path Sum
Binary Tree Maximum Path Sum
A path in a binary tree is a sequence of nodes where each pair of adjacent nodes in the sequence has an edge connecting them. A node can only appear in the sequence at most once. Note that the path does not need to pass through the root.
The path sum of a path is the sum of the node's values in the path.
Given the root
of a binary tree, return the maximum path sum of any non-empty path.
Example 1:
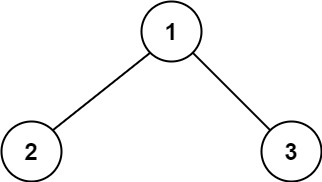
Input: root = [1,2,3]
Output: 6
Explanation: The optimal path is 2 -> 1 -> 3 with a path sum of 2 + 1 + 3 = 6.
Example 2:
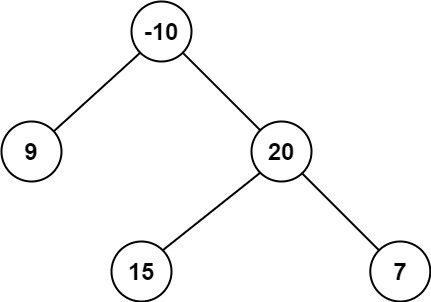
Input: root = [-10,9,20,null,null,15,7]
Output: 42
Explanation: The optimal path is 15 -> 20 -> 7 with a path sum of 15 + 20 + 7 = 42.
Constraints:
- The number of nodes in the tree is in the range
[1, 3 * 104]
. -1000 <= Node.val <= 1000
The Max Path from this node
const maxPathSum = (root) => {
let max = -Infinity;
const findSums = (node) => {
// Base case / hit a null
if (!node) return 0;
let left = findSums(node.left),
right = findSums(node.right),
allSum = left + right + node.val,
leftNodeSum = left + node.val,
rightNodeSum = right + node.val;
// Max is all possible combinations
max = Math.max(max, node.val, allSum, leftNodeSum, rightNodeSum);
// Return the MAX path, which can be node.val, left + node.val, or right + node.val
return Math.max(leftNodeSum, rightNodeSum, node.val);
};
findSums(root);
return max;
};
var maxPathSum = function(root) { var max = -Number.MAX_VALUE; getMaxSum(root); return max; function getMaxSum(node) { if (!node) return 0; var leftSum = getMaxSum(node.left); var rightSum = getMaxSum(node.right); max = Math.max(max, node.val + leftSum + rightSum); return Math.max(0, node.val + leftSum, node.val + rightSum); } };
Conclusion
That’s all folks! In this post, we solved LeetCode problem 124. Binary Tree Maximum Path Sum
I hope you have enjoyed this post. Feel free to share your thoughts on this.
You can find the complete source code on my GitHub repository. If you like what you learn. feel free to fork 🔪 and star ⭐ it.
In this blog, I have tried to collect & present the most important points to consider when improving Data structure and logic, feel free to add, edit, comment, or ask. For more information please reach me here
Happy coding!
Comments
Post a Comment